A couple of weeks ago I attended a workshop on Arduino at Local Motors (LM). There were about 20 people, some with experience; others with none. Some brought their own Arduino kits and LM provided kits for us who did not have. First, there was a presentation on Arduino so that we could understand the basics. Then we started the workshop; our first exercise was to blink a LED. In Arduino terms, you enable one pin as output, then you loop to set the value to 0 and 1. Here’s the code:
// Pin 13 has a LED connected on most Arduino boards.
int led = 13;
// the setup routine runs once when you press reset:
void setup() {
// initialize the digital pin as an output.
pinMode(led, OUTPUT);
}
// the loop routine runs over and over again forever:
void loop() {
digitalWrite(led, HIGH); // turn the LED on (HIGH is the voltage level)
delay(1000); // wait for a second
digitalWrite(led, LOW); // turn the LED off by making the voltage LOW
delay(1000); // wait for a second
}
I installed the Arduino IDE (version 1.6.4) on my laptop (14.04) sudo apt-get install arduino arduino-core
so I could interface with the board which just connects to a USB port. LM provided breadboards so we could try various schematics, blink 2 LEDs with variable timing, control motors, etc. The LM people were very helpful. I plan to go to the next workshop, it was fun.
_____
http://www.arduino.cc/
https://localmotors.com/explore/search/arduino/
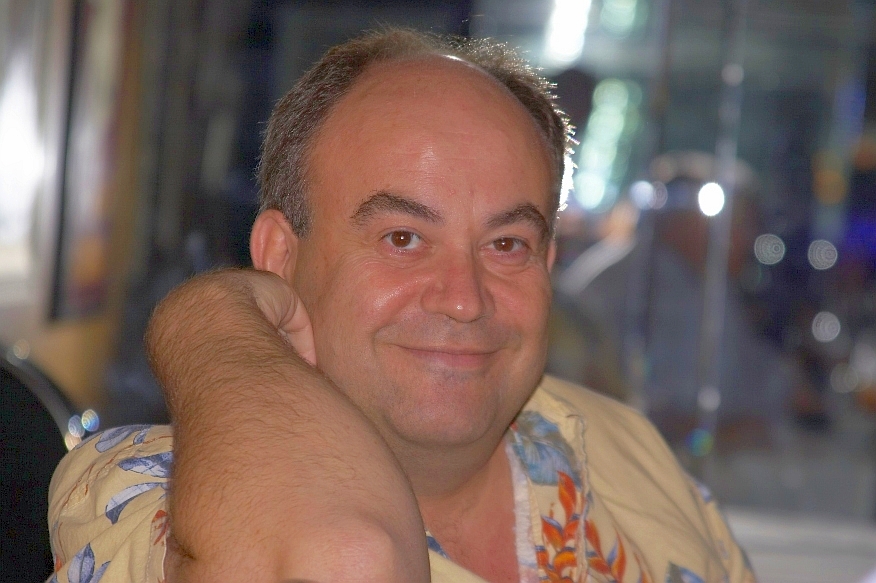